C Program to Find the Sum of Natural Numbers using Recursion
Example to find the sum of natural numbers by using a recursive function.
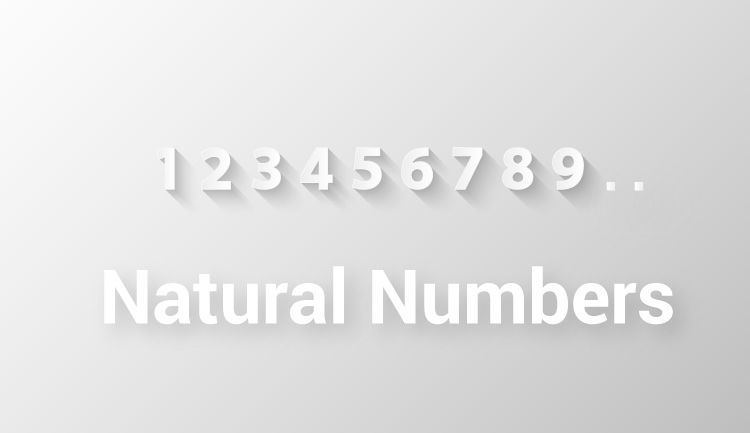
The positive numbers 1, 2, 3... are known as natural numbers. The program below takes a positive integer from the user and calculates the sum up to the given number.
You can find the sum of natural numbers using loop as well. However, you will learn to solve this problem using recursion here.
The positive numbers 1, 2, 3... are known as natural numbers. The program below takes a positive integer from the user and calculates the sum up to the given number.
You can find the sum of natural numbers using loop as well. However, you will learn to solve this problem using recursion here.
Example: Sum of Natural Numbers Using Recursion
#include <stdio.h>
int addNumbers(int n);
int main()
{
int num;
printf("Enter a positive integer: ");
scanf("%d", &num);
printf("Sum = %d",addNumbers(num));
return 0;
}
int addNumbers(int n)
{
if(n != 0)
return n + addNumbers(n-1);
else
return n;
}
Output
Enter a positive integer: 20
Sum = 210
Suppose the user entered 20.
Initially, the addNumbers()
is called from the main()
function with 20 passed as an argument.
The number (20) is added to the result of addNumbers(19)
.
In the next function call from addNumbers()
to addNumbers()
, 19 is passed which is added to the result of addNumbers(18)
. This process continues until n is equal to 0.
When n is equal to 0, there is no recursive call and this returns the sum of integers to the main()
function.
#include <stdio.h>
int addNumbers(int n);
int main()
{
int num;
printf("Enter a positive integer: ");
scanf("%d", &num);
printf("Sum = %d",addNumbers(num));
return 0;
}
int addNumbers(int n)
{
if(n != 0)
return n + addNumbers(n-1);
else
return n;
}
Output
Enter a positive integer: 20 Sum = 210
Suppose the user entered 20.
Initially, the
addNumbers()
is called from the main()
function with 20 passed as an argument.
The number (20) is added to the result of
addNumbers(19)
.
In the next function call from
addNumbers()
to addNumbers()
, 19 is passed which is added to the result of addNumbers(18)
. This process continues until n is equal to 0.
When n is equal to 0, there is no recursive call and this returns the sum of integers to the
main()
function.
No comments:
Post a Comment